In the previous lesson, OAuth 2.0: A Secure Framework for Modern Applications, we explored how OAuth 2.0 improves security by allowing users (resource owners) to delegate specific permissions to third-party applications (clients) without sharing their passwords.
But how do we determine what resources a client can access and what actions they’re allowed to perform?
That’s where OAuth 2.0 scopes come into play.
Master OAuth 2.0: A Practical Guide to API Security
Join 9,000+ students in the
🔥 Highest-Rated OAuth 2.0 Course on Udemy
🔐 Deep-dive into flows, tokens, and advanced security extensions
🧠 Real-world scenarios, attacker simulations & decision trees
🏗️ Designed for developers, architects & security pros
OAuth 2.0 scopes are key to implementing secure, fine-grained access control in modern API ecosystems. A well-designed scope strategy not only limits client access to exactly what’s necessary—it also enhances user transparency by clearly communicating which data and actions an application is requesting.
In this article, we’ll start by exploring practical strategies for identifying the scopes your application truly needs. Then we’ll dive into best practices for structuring, naming, and managing those scopes effectively. Specifically, we’ll explore:
- What OAuth 2.0 scopes are and why they matter for API security.
- Strategies for identifying and selecting appropriate OAuth 2.0 scopes.
- Best practices for structuring, naming, and maintaining OAuth 2.0 scopes clearly and securely.
By the end, you’ll have a clear understanding of how to design and manage OAuth 2.0 scopes to build secure, scalable, and user-friendly API authorization models.
What Are OAuth 2.0 Scopes — and Why Do They Matter?
OAuth 2.0 scopes define precisely which resources a third-party client application can access on behalf of a user, as well as what actions it can perform on those resources. OAuth scopes essentially act as gatekeepers, enforcing explicit permissions and ensuring that client applications receive only the minimum access necessary.
When a third-party application (such as Canva) requests access to your data, you typically see a consent screen detailing exactly what information or resources it needs. These requests generally consist of clearly defined categories, such as:
- Resources: Your profile information (name, email address, profile picture), calendar events, documents, photos, or contacts.
- Actions: Reading, modifying, deleting, or sharing this information.
For example, the following screenshot illustrates Canva requesting specific permissions from a Google account. Here, Google clearly outlines what data Canva is requesting access to (name, email address, language preference, and profile picture), allowing users to make an informed decision about granting access:

“Canva requesting permission to access user information from a Google account, demonstrating OAuth 2.0 scopes clearly listed during the consent process.”
By explicitly defining scopes in this way, we implement robust, fine-grained access control aligned with the principle of least privilege—ensuring applications only request and receive the exact access they need, enhancing both security and user transparency.
Benefits of Proper OAuth Scope Management
- Minimal Access Permissions: Clients receive only the exact permissions they need—no more, no less. This enforces the Principle of Least Privilege, reducing the attack surface and ensuring damage is limited even if a token is compromised.
- Compliance & Auditing: OAuth 2.0 scopes help meet regulatory requirements (e.g., GDPR, HIPAA) by enabling strict access control, and they support auditability by making it easy to track and log who has access to what.
- Enhanced User Transparency: Scopes provide clear descriptions during consent prompts, helping users make informed decisions about the permissions they grant—building trust and reducing friction.
- Granular Delegation: Scopes allow users to delegate access to specific resources and operations without exposing their entire account, enabling secure and contextual third-party integrations.
- Developer & API Management Benefits: Well-defined scopes simplify integration for developers and make it easier for API providers to manage permissions across applications and user roles.
- Ease of Token Validation: Since scopes are typically embedded in access tokens, resource servers can efficiently enforce permissions without needing constant checks against the authorization server.
- Cross-Platform Access Control Consistency: OAuth 2.0 scopes provide a unified authorization model that works seamlessly across web apps, mobile apps, and backend systems. This allows you to define and enforce permissions once and apply them consistently, regardless of the platform the client is running on.
- Scalable & Future-Proof Authorization: Scopes support modular, evolving permission strategies. With versioning and logical naming, you can grow your system over time without breaking existing clients.
Strategies for Determining OAuth 2.0 Scopes
Designing effective OAuth 2.0 scopes begins with a deep understanding of your application’s domain, user roles, workflows, and compliance requirements. The goal at this stage isn’t to name or structure scopes yet—but to identify what permissions your application truly needs and why.
There’s no one-size-fits-all strategy, but several well-established approaches can help you make informed decisions. These strategies, often used in combination, provide a solid foundation for building a secure, scalable, and purpose-driven scope model.
1. Business-Aligned Scopes
Aligning OAuth scopes directly with your core business processes ensures intuitive permissions, making them easily understood by both technical and non-technical stakeholders. Business-aligned scopes naturally reflect real-world organizational activities and align closely with internal workflows, reducing the learning curve for developers and simplifying permission management.
Example OAuth scopes:
– Allows viewing customer orders.orders.view
– Enables creating, updating, or deleting products.products.manage
– Grants access to customer information.customers.read
A prominent example of this method can be seen in Microsoft Graph API, which aligns its OAuth 2.0 scopes directly with everyday business activities, such as managing calendars (
) or sending emails Calendars
.Read(
). This alignment simplifies integration by clearly communicating what permissions an application requires based on common organizational tasks
.SendMail
2. Role-Based Access Control (RBAC)
With Role-Based Access Control, scopes are defined and grouped according to specific roles within your organization or application. Permissions correspond directly to job functions or user responsibilities, making it especially valuable for large organizations, multi-tenant systems, or applications with complex permission structures.
RBAC significantly simplifies the administration of scopes by allowing permissions to be managed at the role level rather than individually per user or client. As roles evolve or responsibilities shift, updating access becomes both efficient and manageable.
Example RBAC scopes:
- Admin – Comprehensive access to all scopes.
- Support Agent– Limited permissions like
andorders.read
customers.read
- Sales Manager – Permissions for sales tasks such as
andleads.read
leads.write
This approach is utilized effectively by platforms such as Microsoft 365, which defines clear administrative roles like Exchange Administrator, SharePoint Administrator, and Global Administrator. Each role is associated with specific permissions clearly aligned with their responsibilities, enabling easy management and scalability.
3. Audit and Compliance-Driven Scopes
Industries such as healthcare (regulated by HIPAA), finance, or government frequently require detailed logging and strict tracking of user actions. In such environments, scopes are often broken down to granular levels, enabling precise auditing and regulatory compliance.
Instead of broad permissions like
, scopes are explicitly defined for specific audited actions:products.write
– Specifically logs creation of new products.products.add
– Explicitly tracks modifications to existing products.products.update
However, it’s important to balance granularity with complexity. If detailed audit logging satisfies compliance without necessitating highly granular scopes, consider consolidating related permissions (like
) to reduce management overhead. Always weigh compliance demands against maintainability.products.write
Now that we’ve explored how to identify the right scopes for your application, we’ll shift our focus to how those scopes should be structured and named.
Best Practices for Structuring and Naming OAuth 2.0 Scopes
Once you’ve identified the scopes your application needs, the next step is to define them in a way that’s secure, consistent, and easy to manage. Structuring and naming OAuth 2.0 scopes isn’t just about clarity—it directly impacts developer experience, maintainability, and overall system security.
In this section, we’ll explore five best practices that will help you create an effective and professional scope strategy—one that supports long-term scalability while remaining intuitive for developers and end users.
1. Find the Right Level of Granularity
Finding the right level of detail in your OAuth scopes is one of the most important design decisions you’ll make.
On one hand, using scopes that are too broad—like
—might seem convenient at first. But this kind of scope gives clients excessive power, increasing the risk of accidental or malicious misuse.products.manage
On the other hand, going too narrow—defining individual scopes like
, products.create
products.update
, and products.delete
—can result in an overwhelming number of scopes that are difficult to manage, assign, and understand.
The key is to strike a balance. A common and effective approach is to separate read and write operations, such as
and products.read
. This gives you enough control without overcomplicating your access model. When compliance or auditing needs arise, you can introduce more granular scopes as needed—but avoid “scope explosion” unless there’s a clear justification.products.write
2. Enforce the Principle of Least Privilege
One of the core security principles you should apply is least privilege—only granting the minimum access necessary to perform a specific task.
For example, if a client application only needs to read order data, it should be granted
, not orders.read
. This reduces the attack surface and limits the potential damage if a token is ever compromised.orders.write
Least privilege also improves user confidence during the consent process. Users are more likely to approve access requests that are specific and appropriately scoped, rather than overly broad and invasive ones.
3. Use Consistent and Hierarchical Naming Conventions
Clear, structured naming is essential for long-term maintainability. As your API grows, inconsistent or unclear scope names will quickly become a source of confusion and mistakes.
A hierarchical naming convention provides structure and improves clarity. Scopes should follow a logical format that reflects both the resource and the level of access.
Example:
— Allows viewing order data only (e.g., order history, status, details)orders.read
— Grants permission to create new orders or update existing onesorders.write
— Full control over orders, including deletion or administrative overridesorders.admin
You can further standardize your naming across services by using a format like:
— e.g.,resource.action
users.read
,payments.write
— e.g.,module.resource.action
inventory.products.write
This consistency makes it easier for developers to understand and predict scope behavior, and for security teams to audit and review permissions across the system.
Real-World Example: Microsoft Graph
Microsoft Graph exemplifies excellent hierarchical naming practices in their OAuth 2.0 scope definitions. Consider these scopes:
— Allows the app to read, create, update, and delete only files that belong to or are shared with the signed-in user.Files.ReadWrite
— Extends this capability, granting the app access to all files within the organization accessible by the signed-in user, including shared files and team sites.Files.ReadWrite.All
Microsoft’s approach clearly communicates scope permissions, adheres to logical naming patterns, and effectively supports both developers and security teams in understanding and managing permissions.
4. Manage OAuth Scope Changes Gracefully
As your API evolves, you might need to update or refine the permissions associated with your OAuth 2.0 scopes. For example, you may decide to adjust the behavior of an existing scope like
to enhance security, support new business requirements, or comply with updated regulations.orders.write
However, directly changing the permissions of an existing scope can disrupt applications currently relying on that scope’s original behavior. To avoid causing breaking changes and potentially affecting user experience, it’s often safer and clearer to introduce new, descriptive scopes rather than altering existing ones.
– Original scope, maintains previous permissions (e.g., general order viewing).orders.read
– New scope offering enhanced access with more detailed order information.orders.read.detailed
This approach ensures that existing clients continue to operate smoothly using their current scopes, while newer or updated clients can opt into enhanced permissions by adopting newly introduced scopes.
Real-World Example: Google’s OAuth 2.0 Scopes
Google provides a practical example of managing OAuth 2.0 scopes effectively. Instead of modifying existing scopes—which could disrupt thousands of existing integrations—they introduce additional scopes alongside original ones to offer developers more granular, precise permissions.
Consider Google Calendar’s OAuth 2.0 scopes:
https://www.googleapis.com/auth/calendar
Provides full read and write access to the user’s calendars, including creating, updating, and deleting events.https://www.googleapis.com/auth/calendar.readonly
Allows read-only access to the user’s calendars, enabling viewing events without modification capabilities.https://www.googleapis.com/auth/calendar.events.readonly
Further restricts permissions specifically to viewing events, without access to other calendar details or the ability to modify anything.
By introducing new, more specific scopes rather than modifying existing ones, Google maintains stable, backward-compatible integrations for millions of existing apps, while enabling new applications to adopt more restrictive permissions aligned with best security practices.
Benefits of Introducing New OAuth Scopes:
- Stability: Avoids disrupting existing client integrations.
- Clear Communication: Explicitly describes the permissions each scope grants, improving understanding for developers and end-users.
- Smooth Migration: Allows existing clients to gradually transition to updated scopes at their convenience.
5. Provide Clear, Contextual Documentation
Even the best scope design can fall flat without good documentation. Both developers and users need to understand what each scope does, and why it’s being requested.
- For developers: Include detailed technical documentation—what endpoints are associated with each scope, what actions are permitted, and what security context is required. This prevents misuse and accelerates integration.
- For users: Make consent experiences transparent by providing simple, human-readable descriptions. For example, instead of “Access to calendar data,” say: “Allows this app to view your upcoming events.”
Clear documentation builds trust, reduces friction during authorization, and ensures that your scopes are used properly.
Final Thoughts
OAuth 2.0 scopes are fundamental to securing modern APIs. By carefully identifying permissions, clearly structuring scopes, and consistently managing them, you strengthen security, simplify developer integration, and foster user trust. Adopting these strategies and best practices positions your API for long-term scalability, compliance, and sustainable growth.
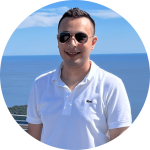
Muhannad Darraj
Senior Software Engineer @ Adesso and instructor of the highest-rated OAuth 2.0 course on Udemy.
I specialize in secure application architectures, OAuth 2.0, OpenID Connect, and Zero Trust. I’m passionate about helping developers and architects design secure, scalable APIs through hands-on learning and real-world scenarios. Want to dive deeper? Check out my course below 👇
Master OAuth 2.0: A Practical Guide to API Security
Join 9,000+ students in the
🔥 Highest-Rated OAuth 2.0 Course on Udemy
🔐 Deep-dive into flows, tokens, and advanced security extensions
🧠 Real-world scenarios, attacker simulations & decision trees
🏗️ Designed for developers, architects & security pros